Enhance Your Python Coding Skills With These Top 10 Data Structures
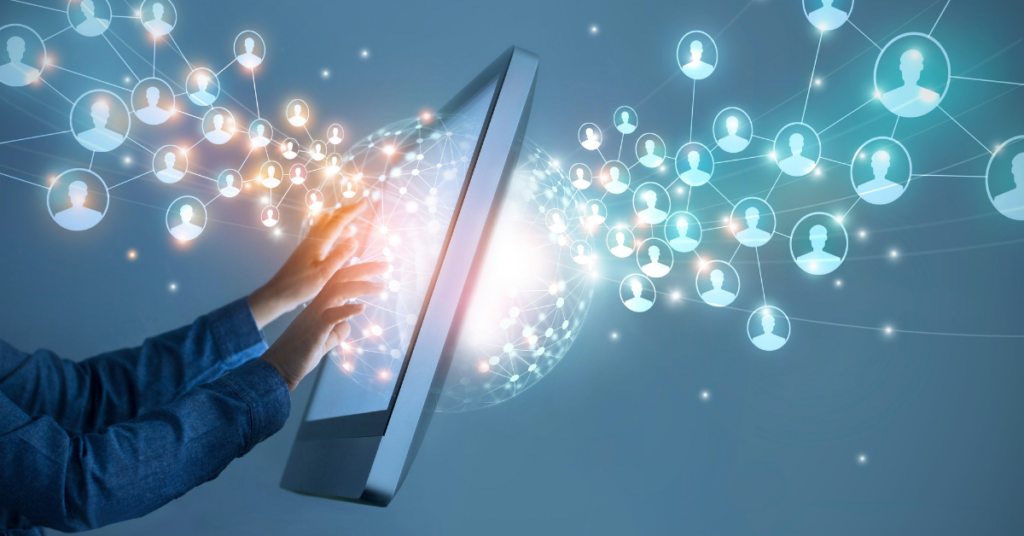
Data structures are the cornerstone of efficient coding in programming, especially in Python. Understanding these structures becomes crucial for any developer writing robust and scalable code. Furthermore, Python offers a variety of data structures, each designed to tackle specific problems and scenarios in the most efficient way possible. This guide delves into the most common Python data structures, exploring their unique characteristics and practical applications.
What are Python Data Structures?
Python data structures are fundamental ways of organizing and storing data. They include built-in types like lists, tuples, and dictionaries, providing various data management methods. Furthermore, these structures optimize data access and manipulation, enhancing programming efficiency and performance. Finally, they form the backbone of Python programming, facilitating data handling in a structured and efficient manner.
Here’s a list of various Python data structures that can be implemented for data processing efficiency, improved memory management, and a well-organized coding experience.Â
1. ListsÂ
Python lists are mutable sequences that allow for dynamic data storage, offering versatility in indexing and a range of operations, including adding, removing, and sorting elements. Furthermore, their flexibility makes them ideal for numerous programming scenarios.
# Creating a listfruits = [“apple”, “banana”, “cherry”]
# Adding elements fruits.append(“orange”) # Removing elements fruits.remove(“banana”) # Sorting the list fruits.sort() |
2. TuplesÂ
Unlike lists, Python tuples are immutable. This means that once defined, their elements can’t be changed. For this reason, they are perfect for storing data that should remain constant throughout the life of a program.
# Creating a tuplecoordinates = (3.0, 4.0)
# Accessing tuple elements x, y = coordinates |
3. ArraysÂ
Arrays in Python, while similar to lists, focus on storing elements of a single data type. Consequently, this makes them more memory efficient and suitable for large data sets where performance is critical.
from array import array# Creating an array of integers
numbers = array(‘i’, [1, 2, 3, 4]) # Accessing array elements first_number = numbers[0] |
4. Strings
Python strings are immutable sequences of Unicode or fixed-length characters and are essential for text manipulation. Furthermore, their operations make handling and transforming text data straightforward.
# Creating a stringgreeting = “Hello, World!”
# Accessing string characters first_char = greeting[0] # Slicing a string hello = greeting[:5] |
5. SetsÂ
Sets in Python are unindexed collections of unique elements. Moreover, their hashable nature allows for fast membership testing and eliminating duplicate entries. This thus makes them ideal for certain data processing tasks.
# Creating a setunique_numbers = {1, 2, 3, 4, 4, 5}
# Adding an element to the set unique_numbers.add(6) # Removing an element from the set unique_numbers.discard(3) |
ALSO READ: Why is it Important to Learn Python in Data Science?
6. DictionariesÂ
Python dictionaries store data in key-value pairs. Equally important, this makes them incredibly efficient for retrieving data and ideal for crucial relationships between elements.
# Creating a dictionaryages = {“Alice”: 25, “Bob”: 30}
# Accessing dictionary values age_of_alice = ages[“Alice”] # Adding a new key-value pair ages[“Charlie”] = 28 |
7. StacksÂ
Stacks in Python operate on the Last In, First Out (LIFO) principle. For this reason, they are widely used in algorithm implementations such as undo mechanisms.
stack = []# Pushing an item to the stack
stack.append(‘a’) stack.append(‘b’) # Popping an item from the stack top = stack.pop() |
8. QueuesÂ
Queues in Python, operating on the First In, First Out (FIFO) principle, are essential in scenarios like task scheduling.
from collections import dequequeue = deque()
# Enqueueing queue.append(‘first’) queue.append(‘second’) # Dequeueing first = queue.popleft() |
9. HeapsÂ
Python’s binary heaps, especially min heaps, offer an efficient way to constantly access the smallest element, pivotal in algorithms like priority queues.
import heapq# Creating a heap
heap = [3, 1, 4, 1, 5, 9, 2, 6, 5] heapq.heapify(heap) # Accessing the smallest element smallest = heap[0] |
10. Priority QueuesÂ
Python’s priority queues, implemented using the heapq module, allow elements to be processed based on their priority. Hence, they are essential in algorithms where order of processing is critical.
import heapq# Creating a priority queue
pq = [] # Adding elements heapq.heappush(pq, (2, ‘code’)) heapq.heappush(pq, (1, ‘eat’)) heapq.heappush(pq, (3, ‘sleep’)) # Popping an element based on priority next_task = heapq.heappop(pq) |
How Can Python Data Structures Improve Data Manipulation Tasks?
In the world of coding, Python data structures are fundamental. Furthermore, they offer organized and efficient ways of storing and manipulating data. Moreover, these structures, such as Python list, Python tuples, Python sets, and Python dictionaries, provide various methods of data arrangement. As a result, they cater to different needs and scenarios in data handling.Â
Additionally, the versatility of Python data structures extends to more specialized types, such as Python linked list, which are essential in certain algorithm implementations. Equally important are Python list and Python dictionaries, which are widely used due to their flexibility and efficiency in data manipulation. Therefore, a deep grasp of Python data structures, including Python tuple and Python sets, is invaluable in solving many programming challenges. Hence, Python data structures form the backbone of effective and efficient code in numerous applications across diverse fields.
ALSO READ: Here’s Why Use Python for Data Science
Which Python Data Structure is Best for Storing a Collection of Objects?
When it comes to storing collections, Python lists and Python sets are popular choices. Furthermore, a Python list allows storing an ordered sequence of items, which can be varied. Therefore, they are incredibly versatile. Again, Python sets are helpful, too, particularly for maintaining unique elements and offering fast operations for checking membership.
Largely, the choice between a Python list and a Python set depends mainly on the specific requirements of the task at hand. Moreover, Python data structures like Python tuples and Python dictionaries also play a crucial role. For instance, a Python tuple, being immutable, is ideal for storing fixed data, thus offering stability in your programs. Additionally, a Python dictionary is invaluable for mapping unique keys to values, making data retrieval efficient.Â
Consequently, the application of these Python data structures, including Python linked lists, varies based on the complexity and nature of the data involved. Moreover, the adaptability of the data structures, like the Python linked list, allows for more complex data handling, such as in linked data scenarios. Therefore, each Python data structure, be it a Python list, Python set, Python tuple, or Python dictionary, finds its unique place in a coder’s toolkit. This is the reason for its widespread popularity and application in various programming domains.
ALSO READ: Staying Current in the Evolving World of Data Analysis: Top 8 Tools For Success
What are the Advantages of Using a Python Dictionary Over a List?
A Python dictionary, another integral Python data structure, stands out for its key-value storage format. Some of the benefits of using a Python dictionary over a list are as follows:
- This format enables rapid data retrieval, outperforming Python lists in speed
- Furthermore, data in dictionaries can be accessed swiftly using keys, unlike lists, which may need element iteration
- Additionally, in scenarios demanding quick data access, Python dictionaries are typically more efficient
How Can Python Sets be Applied in Real-World Scenarios?
Unique among Python data structures, Python sets are perfect for scenarios where the uniqueness of elements is paramount. Furthermore, they are commonly used in data analysis for tasks like removing duplicate entries. Consequently, Python sets are invaluable in situations requiring the handling of large data sets to ensure data integrity and efficiency.Â
Therefore, their application extends to many real-world scenarios, including database operations and data preprocessing in machine learning.
Additionally, other Python data structures like Python lists, Python tuples, and Python dictionaries also play vital roles in various programming contexts. For instance, a Python list is ideal for maintaining an ordered collection of items, making it a go-to choice for many developers. Moreover, Python tuples, being immutable, offer a reliable way to store unchangeable data, which is crucial in specific algorithmic processes. Also, Python dictionaries provide a highly efficient means of data access through key-value pairings.Â
Lastly, Python-linked lists are essential when data elements need to be frequently inserted or removed, showcasing the flexibility and utility of Python data structures in complex coding solutions.
ALSO READ: Top 13 Python Interview Questions and Answers to Crack Your Next Python Job
A solid grasp of Python data structures is essential for aspiring or seasoned developers. Therefore, it’s critical to understand each structure’s nuances to enhance the efficiency of the code. The best way to upgrade your skills is with Emeritus’ data science courses. They will help deepen your understanding and application of these fundamental concepts.
Write to us at content@emeritus.org