Pattern Program in Python: Here’s What You need to Know
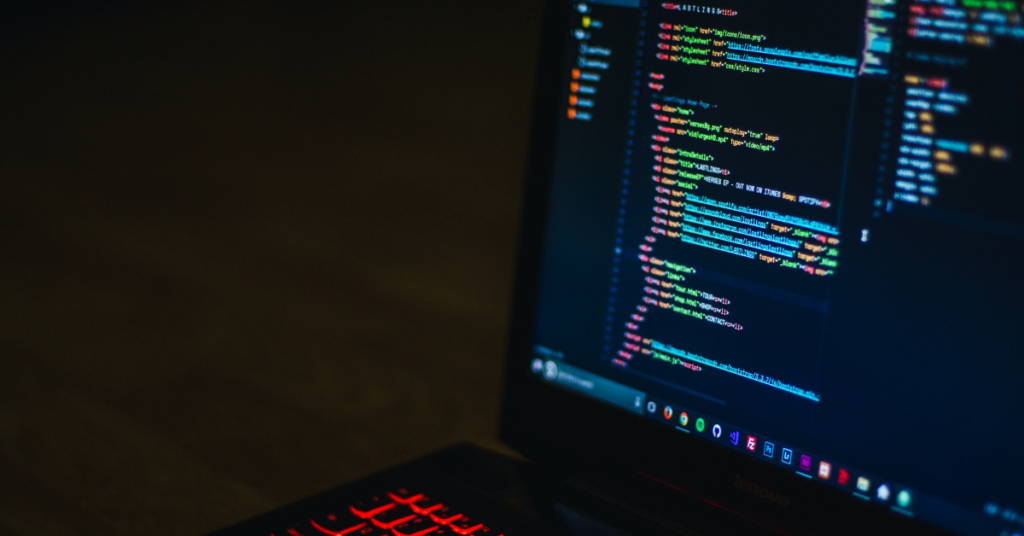
Whether professional or tech-enthusiast, there can be no denying that Python is at the tip of everyone’s mouth. Why? Primarily because of its simplicity, scalability, rich libraries, and extreme diversity in terms of application. Whether it’s the Netflix algorithm that recommends shows or movies you watch or designing software for autonomous cars—Python is used everywhere. Now, one way to master this language is by learning how to create patterns using Python. This blog will introduce you to several interesting pattern program in Python, providing examples and explanations to help you better understand and implement these patterns.
What is Python?
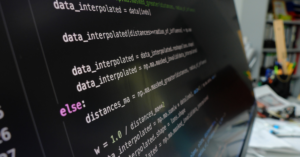
Before we dive into creating patterns, let’s briefly explore what Python is. Python is an open-source, general-purpose, object-oriented programming language that was first introduced by Guido van Rossum in the late 1980s. In short, this language was designed with simplicity in mind, emphasizing code readability and ease of writing. Python’s syntax is intuitive, often resembling plain English. For instance, if you want to print “good morning, everyone” in Python, the code would be:
print(“good morning, everyone”)
Yes, that’s how simple Python can be. Consequently, this simplicity reduces the complexity of coding and makes it an excellent choice for those new to programming. Despite its simplicity, it’s used for data analysis, web development, IoT applications, artificial intelligence and machine learning algorithms.
1. Benefits of Python
Using Python is beneficial for a number of reasons:
- Python is clear and easy to understand. Its syntax allows writing code that reads almost like English
- Python allows both objective oriented and procedural coding
- Python offers a vast collection of libraries that simplify complex tasks(i.e.,web development, data analysis, etc)
- Python is extremely versatile, capable of running on a diverse range of operating systems such as Windows, macOS, and Linux
- Python’s simplicity allows for faster development cycles, which in turn enables quick prototyping and testing
- Python is highly scalable, and is suitable for handling both small and large projects
- Python can easily integrate with other languages and technologies, enhancing its flexibility
ALSO READ: Python Programming for Beginners: All You Need to Know
Pattern Program in Python
A pattern program is a sequence of instructions designed to create a specific visual pattern using numbers, symbols, or characters. These patterns can range from simple shapes such as a pyramid to more complex designs like spiral patterns with numbers. They serve as excellent practice for understanding fundamental programming concepts. Learning to write pattern programs in Python offers several key advantages:
- Pattern programs are an effective way to learn and master loops, including for and while loops
- Creating patterns requires breaking problems down into manageable steps, which improves logical reasoning
- Writing patterns involves creative problem-solving and enhances algorithmic thinking
- Working on pattern programs helps you practice organizing your code in a clear and efficient manner
- You’ll learn how to format output precisely using Python’s print function and end parameters
- Understanding pattern programs provides a strong foundation for tackling more complex algorithms in the future
- Successfully creating patterns boosts confidence and reinforces the skills needed for more advanced programming challenges
Now, let’s look at a select number of pattern program in Python.
1. Inverted Pyramid
This pattern program in Python prints an inverted pyramid with 17 stars at the top, gradually decreasing by 2 stars per row.
n = 17 # Number of stars at the base
for i in range(n, 0, -2): # Decrease by 2 to maintain an odd number of stars in each row
for j in range((n – i) // 2):
print(” “, end=””) # Print leading spaces to center-align the stars
for j in range(i):
print(“*”, end=””)
print()
Here’s what the output of this program looks like:
*****************
***************
*************
***********
*********
*******
*****
***
*
This code generates an inverted pyramid with 17 stars at the base. The outer loop starts from 17 and decreases by 2, ensuring each row has an odd number of stars. The first inner loop handles the spaces for center alignment, while the second inner loop prints the stars for each row. As the loop progresses, the number of stars decreases, creating the inverted pyramid shape.
2. Right Angle Pyramid
This pattern program in Python generates a pyramid with 9 stars at the base.
n = 9 # Base width in terms of stars
for i in range(1, n + 1):
for j in range(0, i):
print(“* “, end=””)
print()
Here’s the output:
*
* *
* * *
* * * *
* * * * *
* * * * * *
* * * * * * *
* * * * * * * *
* * * * * * * * *
This pattern program in Python uses two nested sets for loops. To elaborate, the outer loop runs from 1 to n (which is 9), representing the rows. Now, for each value of i, the inner loop runs from 0 to i, printing stars (*) in each row. As a result, the number of stars in each row increases by one, creating a right-angle pyramid with 9 stars at its base.
ALSO READ: Why is It Important to Learn Python in Data Science?
3. Diamond Pattern with Stars
Here’s the program for creating a diamond pattern with stars. This particular pattern begins with one star at the top, then increases to 15 stars in the middle. It then descends again to 1 star at the bottom.
n = 8 # Number of rows for the upper half, middle row has 15 stars
# Upper half including the middle row
for i in range(1, n + 1):
for j in range(n, i, -1):
print(” “, end=” “)
for j in range(1, i * 2):
print(“*”, end=” “)
print()
# Lower half
for i in range(n – 1, 0, -1):
for j in range(n, i, -1):
print(” “, end=” “)
for j in range(1, i * 2):
print(“*”, end=” “)
print()
The output of this pattern program in Python looks like this:
*
* * *
* * * * *
* * * * * * *
* * * * * * * * *
* * * * * * * * * * *
* * * * * * * * * * * * *
* * * * * * * * * * * * * * *
* * * * * * * * * * * * *
* * * * * * * * * * *
* * * * * * * * *
* * * * * * *
* * * * *
* * *
*
This diamond pattern with 15 stars in the middle is created using two sets of nested loops. The upper half starts with one star and increases to 15 stars in the middle row. The lower half mirrors the upper half and decreases back to one star. The first inner loop centers the stars by printing spaces. The second inner loop handles the number of stars in each row. They create a symmetrical diamond shape.
4. Spiral Pattern
The following code creates a spiral pattern that begins with the number 1 at the top-left corner and spirals inward. The pattern continues until it reaches a number between 49, filling the matrix in a spiral fashion.
n = 7 # Size of the spiral
matrix = [[0] * n for _ in range(n)]
row, col = 0, 0
number = 1
while number <= 49:
# Traverse right
while col < n and matrix[row][col] == 0:
matrix[row][col] = number
number += 1
col += 1
col -= 1
row += 1
# Traverse down
while row < n and matrix[row][col] == 0:
matrix[row][col] = number
number += 1
row += 1
row -= 1
col -= 1
# Traverse left
while col >= 0 and matrix[row][col] == 0:
matrix[row][col] = number
number += 1
col -= 1
col += 1
row -= 1
# Traverse up
while row >= 0 and matrix[row][col] == 0:
matrix[row][col] = number
number += 1
row -= 1
row += 1
col += 1
for row in matrix:
for col in row:
print(str(col).zfill(2), end=” “)
print()
Output of this pattern program in python will be:
01 02 03 04 05 06 07
24 25 26 27 28 29 08
23 40 41 42 43 30 09
22 39 48 49 44 31 10
21 38 47 46 45 32 11
20 37 36 35 34 33 12
19 18 17 16 15 14 13
This spiral pattern is created using a main while loop that controls the overall filling of the matrix. Inside this, there are four nested while loops that handle the movement in four directions: Right, down, left, and up. These loops work together to fill the matrix with numbers in a spiral order. This ensures the pattern starts at the top-left corner and spirals inward until the matrix is completely filled. The loops continue to execute until the desired number is reached in the matrix.
ALSO READ: What are the Python Functions, Modules, and Packages?
In conclusion, Python continues to dominate the world of programming owing to its ease of use, simplicity of syntax, high scalability, and rich library, among other things. These qualities have secured its position as the top programming language in the August 2024 TIOBE index. It holds a remarkable rating of 18.04% in this index. Since Python’s versatility extends from handling simple scripts to complex machine learning algorithms, it has become a highly sought-after skill in the job market. Whether you’re aiming to enhance your coding skills or pursue a career in tech, mastering Python is a crucial step.
Were you fascinated by the pattern program in Python? If yes, and you’re eager to deepen your Python programming skills, consider enrolling in Emeritus’ online data science courses. Delivered by industry experts and backed by Emeritus, they offer comprehensive training in Python and other important data science tools. They help you stand out in today’s competitive job market.
Write to us at content@emeritus.org