9 Most Convenient Ways to Reverse a String in Java
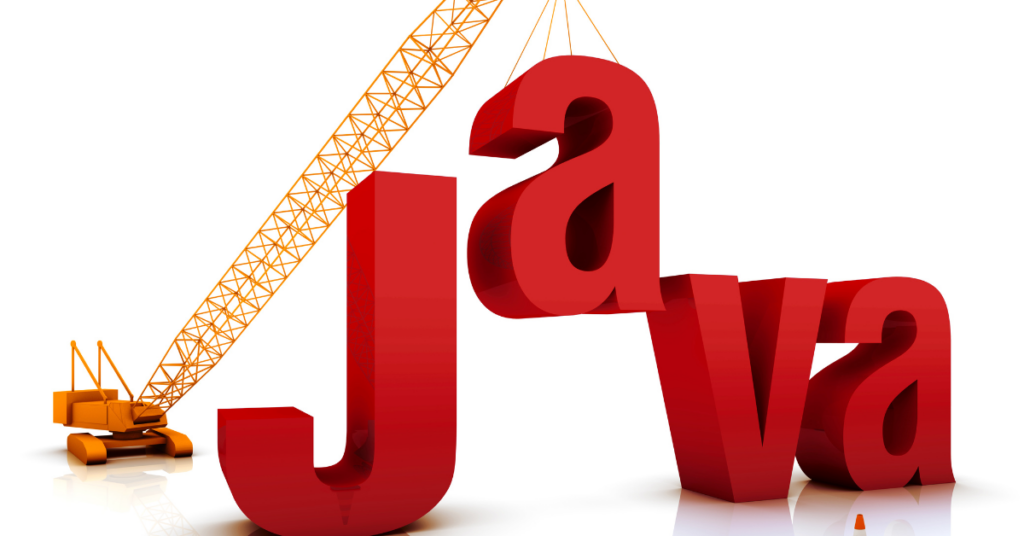
JavaScript is one of the most-used programming languages worldwide. Programmers regularly use strings in Java programming, which is a character array that stores data and acts like an object. Programmers often need to reverse a string in Java for various programming needs. This article discusses the nine most convenient methods to reverse a string in Java.
What is Reverse a String in Java?
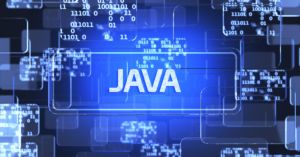
Java programming offers java.lang. The String class is used to create a string object and represent a string using UTF-16. A string is an immutable object in Java programming. In other words, the internal state of a string object remains constant after creation. Therefore, you need to create a new string to reverse an existing string. Programmers can perform various operations using a string. However, reversing a string is one of the most widely used functions in Java programming.
9 Different Ways To Reverse a String in Java
The following are the nine most convenient ways to reverse a string in Java.
1. Creating a New String
Firstly, you must create a new empty string to accommodate the reversed string in this method. Then, you must add each character of the original string in reverse order to the new empty string.
For example,
public class ReverseString
{
Public static String reverse(String str)
{
String reversed = “”;
for(int i = str.length()-1; i>=0; i–)
{
reversed += str.charAt(i);
}
return reversed;
}
public static void main(String[] args)
{
String original= “Hello, World!”;
String reversed= reverse(original);
System.out.printin(“Original string:”+ original);
System.out.printin(“Reversed string:”+ reversed);
}
}
In the above example, the input is the original string ‘Hello, World’. ‘Reversed’ is the new empty string created to accommodate the reversed string. Then, the characters of the original string ‘str’ are added in a reversed order using the ‘+=’ operator. Finally, the code returns and prints the original string and the reversed output ‘!dlroW, olleH’.
2. Using StringBuilder Class
In order to reverse a string using the StringBuilder class in Java, you need to use the built-in reverse() method. String class does not offer a reverse() method. Therefore, you must convert the original string into a StringBuilder class using the append() method.
For example,
String originalstring = “Hello World!”;
Stringbuilder reversedString = new StringBuilder();
reversedString.append(originalString);
reversedString = reversedString.reverse();
System.out.printin(“Original string:”+ originalString);
System.out.printin(“Reversed string:”+ reversedString.toString);
Output:!dlroW olleH
In the above example, first, you need to create a new StringBuilder object named ‘reversedString’. Then you need to add the input string ‘Hello World!’ to it using the ‘append()’ method. Next, you must call the ‘reverse()’ method of the StringBuilder class to reverse the characters of the original string. Lastly, you can print the original and reversed string using the ‘System.out.printIn()’ method.
3. Using a List
You can convert the input string into a list of characters in Java. Then you can reverse that list of characters. Finally, you can convert that list back into a string again.
For example,
import java.util.*;
public class StringReversal
{
public static void main(String[] args)
{
String originalString = “Hello, World!”;
// Convert the string to a list of characters List charList = new Arraylist< > ();
for (char c : originalString.toCharArray())
{
charList.add(c);
}
// reverse the list Collections.reverse(charList);
// Convert the reversed list back to a string StringBuilder reversedString = new StringBuilder(charList.size());
for (Character c: charList)
{
reversedString.append(c);
}
System.out.printin(“Original string:”+ originalString);
System.out.printin(“Reversed string:”+ reversedString.toString);
In the above example, a for-each iterative loop converts the input string into a list of characters. Next, the ‘Collections.reverse()’ method reverses the character list. Lastly, the reversed character list converts back to a string using a StringBuilder class and another iterative for-each loop.
ALSO READ: Here Are 5 Important Reasons to Learn Java Programming in 2024
4. Using a Stack
First, the input string converts into a character array in this method. Then each character of the array pushes into the stack. The stack adds the popped characters to a StringBuilder class to get the reversed string.
For example,
import java.util.*;
public class StringReversal
{
public static void main(String[] args)
{
String originalString = “Hello, world!”;
// Convert the string to a list of characters
List charList = new ArrayList<>();
for (char c : originalString.toCharArray())
{
charList.add(c);
}
// Reverse the list
Collections.reverse(charList);
// Convert the reversed list back to a string
StringBuilder reversedString = new StringBuilder(charList.size());
for (Character c : charList)
{
reversedString.append(c);
}
System.out.println(“Original string: ” + originalString);
System.out.println(“Reversed string: ” + reversedString.toString());
}
}
In the above example, Firstly the original string converts to a character array ‘charList’ using the ‘reverseString()’ method. Then each character of the array pushes into the stack. Next, the ‘Collections.reverse()’ method reverses each character popping out from the stack. Lastly, the reversed character list converts back to a string using a StringBuilder.
5. Using StringBuffer Class
In order to reverse a string using the StringBuffer class in Java, you need to use the built-in reverse() method.
For example,
public class StringBufferExample
{
public static void main(String[] args)
{
String str = “Hello World”;
StringBuffer sb = new StringBuffer(str);
// Reverse the string using StringBuffer
sb.reverse();
// Print the reversed string
System.out.println(“Reversed string: ” + sb.toString());
}
}
In the above example, first, you need to create a new StringBuffer object named ‘new StringBuffer’. Then you need to pass the input string as a parameter to the constructor. Next, you must call the ‘reverse()’ method of the StringBuffer class to reverse the characters of the original string. Lastly, you can print the reversed string using the ‘toString()’ method.
6. Swapping Characters
Using the two pointers technique is the most common approach while using the swapping characters method for string reversal. The first pointer starts from the beginning of the input string, while the second pointer starts from the end of the same string. Next, the pointers exchange the characters pointed by them. The process continues until the pointers move towards each other and finally meet at the midpoint of the string.
For example,
public class StringReverseExample
{
public static void main(String[] args)
{
String input = “Hello, world!”;
char[] charArray = input.toCharArray();
int left = 0;
int right = charArray.length – 1;
while (left < right)
{
char temp = charArray[left];
charArray[left] = charArray[right];
charArray[right] = temp;
left++;
right–;
}
String output = new String(charArray);
System.out.println(output);
}
}
In the above example, the ‘toCharArray()’ method converts the input string into a character array. Then two pointers named ‘left’ and ‘right’ point to the first and last character of the array respectively. Next, the ‘left’ and ‘right’ pointer exchanges their pointed characters using a temporary variable ‘temp’ in the while loop. Lastly, the code prints the original and reversed string using the ‘System.out.printIn()’ method.
7. Using Recursion
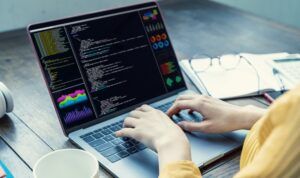
The recursion method of string reversal defines a recursive function that receives the original string as the input. The function returns a reversed string as the output.
For example,
public class StringReversal
{
public static void main(String[] args)
{
String str = “Hello, world!”;
String reversed = reverseString(str);
System.out.printin(reversed);
}
Public static String reverseString(String str)
{
If (str.isEmpty())
{
Return str;
} else
{
return reverseString(str.substring(1)) + str.charAt(0);
}
}
}
In the above example, the ‘reverseString’ function takes the input string argument ‘str’ and returns a reversed string as output. If the original string is empty, the function returns an empty string as output. If the input string is ‘Hello, World!’, the function calls itself recursively using a substring of the input string. The substring excludes the first character of the original string and concatenates the same at the end of the reversed string. The periodic recursive calls continue until the reversed substring is generated.
ALSO READ: Java Programming Language Explained: Everything You Need to Know
8. Using Byte Array
To reverse a string using the byte array method, you need to first convert the input string into a byte array using the ‘getBytes()’ method. Next, you must create another new byte array of equal length to the input byte array. Then copy each byte of the original array and store it reversely in the new byte array. Finally, you have to convert the new byte array into a string again.
For example,
public class ReverseStringExample
{
public static void main(String[] args)
{
String str = “Hello, world!”;
byte[] byteArray = str.getBytes();
byte[] reversedArray = new byte[byteArray.length];
for (int i = byteArray.length – 1, j = 0; i >= 0; i–, j++)
{
reversedArray[j] = byteArray[i];
}
String reversedString = new String(reversedArray);
System.out.println(“Original string: ” + str);
System.out.println(“Reversed string: ” + reversedString);
}
}
In the above example, the input string ‘Hello. World!’ converts into an array of bytes ‘byteArray’ using the ‘getBytes()’ method. Next, the code creates another new byte array of equal length to the original ‘byteArray’. Then each byte of the ‘byteArray’ is copied and stored reversely in the new ‘reversedArray’. Finally, the constructor converts back the reversed byte array into a string by taking it as an argument.
9. Using XOR Operation
This is the least used approach in Java for reversing a string and is not recommended by the experts. However, if you want to use the XOR method, first you must create a new string to XOR with the original string.
For example,
public static String reverseStringUsingXOR(String str)
{
char[] chars = str.toCharArray();
char[] reversedChars = new char[chars.length];
// Reverse the original string
for (int i = 0; i < chars.length; i++)
{
reversedChars[i] = chars[chars.length – i – 1];
}
// XOR each character in the original string with the corresponding character in the reversed string
for (int i = 0; i < chars.length; i++)
{
chars[i] = (char) (chars[i] ^ reversedChars[i]);
reversedChars[i] = (char) (chars[i] ^ reversedChars[i]);
chars[i] = (char) (chars[i] ^ reversedChars[i]);
}
return new String(chars);
}
In the above example, a copy of the original string is created. Next, the copied string is reversed to form the new string to be used for XOR. Finally, each character of the input string XORs with the corresponding characters of the new reversed string.
ALSO READ: The Best 20 Java Interview Questions for Freshers You Should Know About
Programmers prefer a variety of coding languages depending on the type of work they do and their expertise. However, Java is the most widely used programming language for several reasons, including platform independence, object orientation, user-friendliness, free development tools, scalability, security, and robustness. The string is one of the most commonly used data structures in Java programming. Programmers often need to create reverse strings as well. Asking about the methods of creating a reverse string in Java is also a popular question in interviews.
So, are you ready to pursue a career in IT or hone your existing programming skills? Consider enrolling in Emeritus’ IT Courses to take the next step towards a successful career in technology.
Write to us at content@emeritus.org